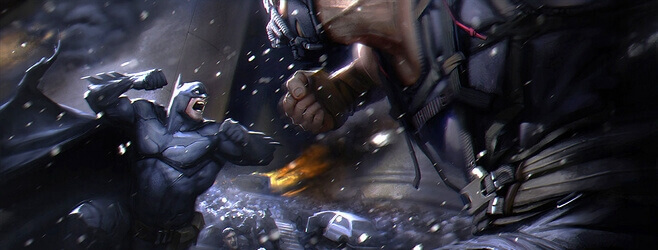
Glide
- coding
- android
- imageloader
Introduction
Glide is a fast and efficient open source media management and image loading framework for Android that wraps media decoding, memory and disk caching, and resource pooling into a simple and easy to use interface.
Glide’s primary focus is on making scrolling any kind of a list of images as smooth and fast as possible, but Glide is also effective for almost any case where you need to fetch, resize, and display a remote image.
You can click here to see more about Glide
.
Import
You are supposed to add the Glide
dependency in the build.gradle
of your module.
compile 'com.github.bumptech.glide:glide:3.7.0'
It’s highly recommended to check the lastest version of Gradle
before you add.
Get started
Glide
is pretty easy to use, you will code like you are writing a sentence!
Glide.with(this)
.load(url)
.into(mImageView);
Or you can simple get a bitmap
with Glide
:
Bitmap bitmap = Glide.with(this)
.load(url)
.asBitmap()
.into(100, 100) //set width and height
.get();
Attributes
Here you can add lots of attributes between load()
and into()
.
placeHolder/error
.placeHolder(R.mipmap.ic_launcher); //display this drawable before downloading.
.error(R.mipmap.ic_launcher); //display this drawable when error occurs while downloading.
crossFade
.crossFade(int duration) //duration from nothing to display your drawable, default value is 300(ms).
thumbnail
.thumbnail(0.1f) //to load a low-quality preview before display the full size image.
resize
.override(100, 100)
position
.fitCenter()
: the short side of the image will fill its parent’s side. (default)
.centerCrop()
: the long side of the image will fill its parent’s side.
cache strategy
.diskCacheStrategy(DiskCacheStrategy.NONE) //cache nothing
.diskCacheStrategy(DiskCacheStrategy.RESULT) //only cache the final image
.diskCacheStrategy(DiskCacheStrategy.SOURCE) //only cache the full size image
.diskCacheStrategy(DiskCacheStrategy.ALL) //cache images in all size(default)
Plugin
Glide
supports many plugins. Here I introduce a libray glide-transformations which focuses on bitmap transforms.
4 examples here:
.bitmapTransform(new CropSquareTransformation(this))
.bitmapTransform(new CropCircleTransformation(this))
.bitmapTransform(new BlurTransformation(this, 5))
.bitmapTransform(new BlurTransformation(this, 25))
Source code
You can check the source code in GlideActivity.java in my another repo.